Building an IAR Development Environment
IAR Systems is a globally renowned provider of embedded system development tools and services. IAR for STM8 is an integrated development environment for STM8 microcontrollers developed by IAR. You can download it from the following link:
https://www.iar.com/products/architectures/risc-v/iar-embedded-workbench-for-stm8/
Creating a Project Using IAR
The installation of IAR is similar to installing regular software and won’t be detailed here. Instead, we’ll focus on how to create an STM8 program project using IAR. First, run the IAR software. The main software interface is shown in the image below.
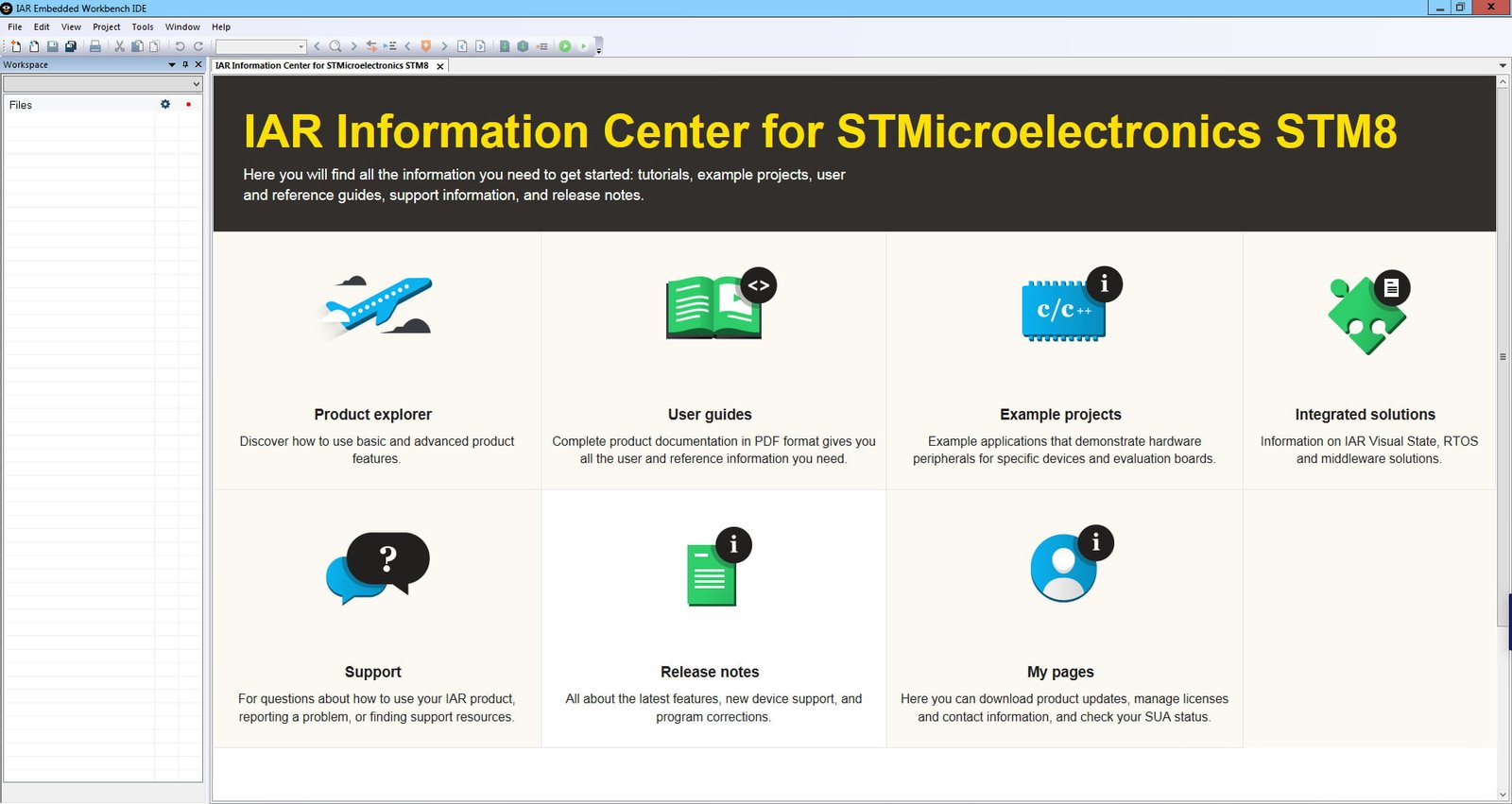
In the toolbar, find the “Project” menu, and in its submenu, select “Create New Project.” A dialog for creating a new project submenu will pop up.
In the project templates, select the C language template. Click the OK button to save the project.
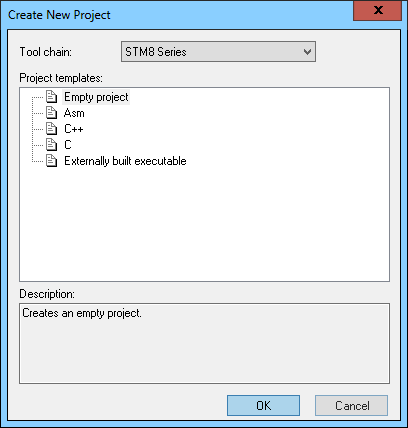
IAR will open the newly created project file as shown below. A new project will automatically create a main.c main program file. Next, you need to configure the project. In the left-side file list pane of the project interface, right-click on the project name and select the “Options” menu from the popup menu, as shown in the image.
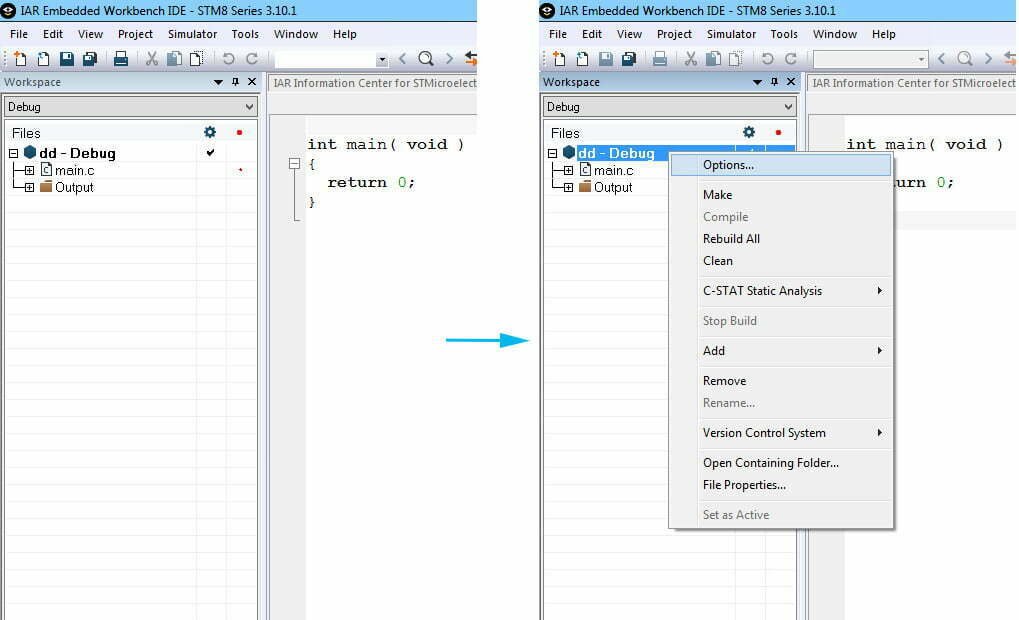
After selecting the “Options” menu, the project properties settings dialog will appear. Choose the first “General Options” property and then the “Target” tab. Select the actual model of the STM8 microcontroller you are using, as shown in the image.
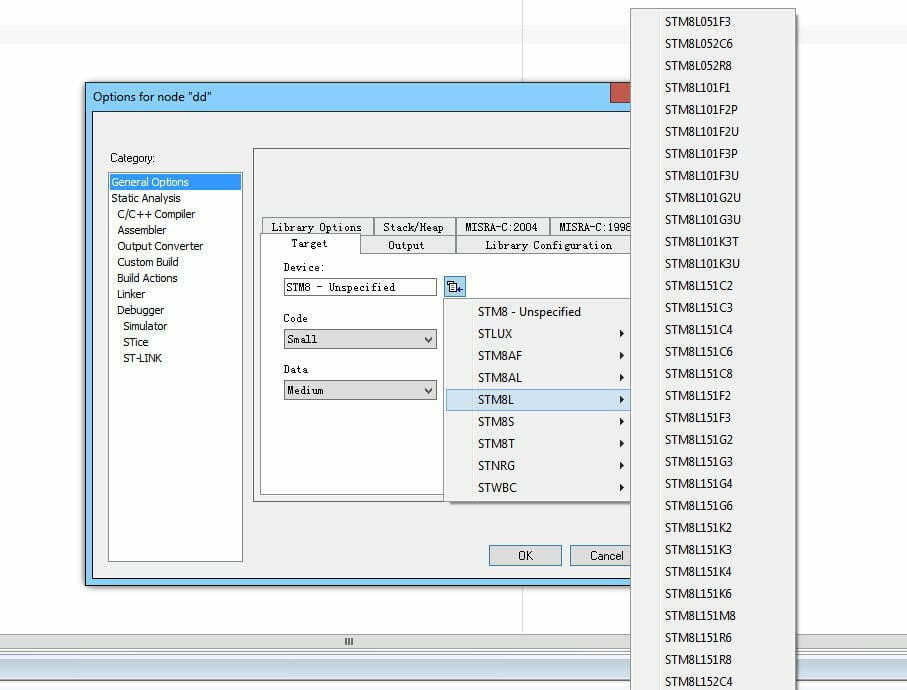
Next, select the “Debugger” property, and the property dialog will change as shown below. In the “Setup” tab, choose “ST-LINK” from the dropdown list in the “Driver” section. Click the OK button to complete the property settings.
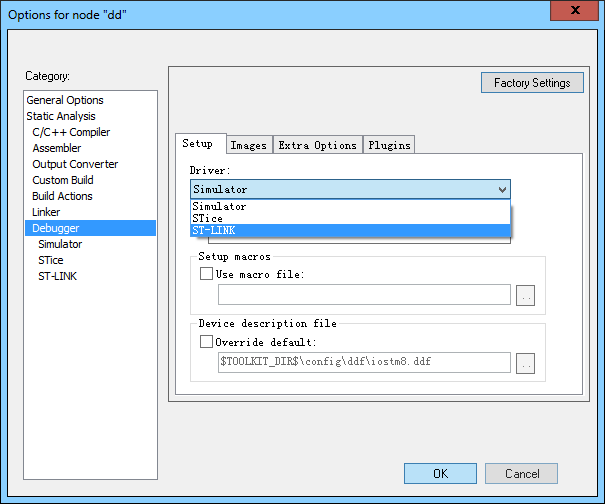
At this point, after compiling the project, you can burn it into the STM8 microcontroller using ST-Link. Specifically, in the IAR software interface toolbar, click the green triangle “download and debug” button to burn the program into the microcontroller. Click the light blue triangle “GO” button to run the program at full speed. Click the red prototype “stop debugging” button with a white cross to exit debugging mode.
Writing Your First Program Using IAR
Although the project above can burn a program into the microcontroller using ST-LINK, it doesn’t contain any substantial operational statements, so the STM8 microcontroller won’t perform any actions. Just as we did in the relevant sections of STVD’s instructions, we can familiarize ourselves with the actual programming steps in IAR by lighting up LED D1.
First, add the STM8 header file to the project. To do this, select the project name in the project file list, right-click, and choose “Add” from the popup menu. Click “Add Files” in the submenu. Next, IAR software will navigate to the STM8/INC directory under the IAR installation directory. Here, you can see files with names like “IOSTM8S105C6.h,” which are header files for various STM8 chips.
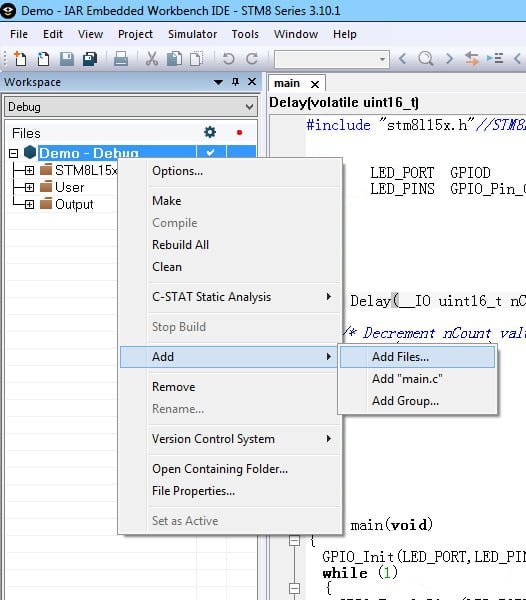
Once added, we also need to include the header in the program using the following reference statement:
#include "IOSTM8S105C6.h"
After including the header file, you can write a program to manipulate GPIO. The program code is the same as the code developed in the previous sections for STVD development. The complete code is as follows:
#include "IOSTM8S105C6.h"
void main(void)
{
PC_DDR |= 0x08; // Set PC3 as output mode
PC_CR1 |= 0x08; // Set PC3 as push-pull output
PC_CR2 |= 0x00; // Set PC3 as 10MHz fast output
PC_ODR ^= 0x08; // Set PC3 output logic low
while (1)
{
}
}
After writing this code, compile and link it, and then use ST-LINK to burn it into the microcontroller. You will observe LED D1 blinking.