The STM8S105K4 microcontroller, known for its efficiency and versatility, is a solid choice for embedded applications in consumer electronics and industrial systems. This article provides an overview of its key features, pin configuration, and block diagram, along with a step-by-step guide to setting up a project in IAR Embedded Workbench. With example code for basic GPIO control and instructions on programming and debugging, this guide will help you get started with the STM8S105K4 quickly and effectively.
Overview
The STM8S105K4 is an 8-bit microcontroller from STMicroelectronics, part of the STM8 family. It is designed for a wide range of applications, providing a balance of performance, power efficiency, and affordability. With its advanced peripherals and embedded EEPROM, the STM8S105K4 is suitable for general-purpose control tasks in consumer electronics, industrial systems, and more.
Features and Specifications
- Core: 8-bit STM8 core with Harvard architecture, operating at up to 16 MHz.
- Memory:
- Flash memory: 16 KB
- RAM: 1 KB
- EEPROM: 640 bytes
- Timers:
- 16-bit advanced control timer (TIM1)
- 16-bit general-purpose timer (TIM2)
- 8-bit basic timer (TIM4)
- Communication Interfaces:
- UART, I²C, and SPI interfaces for versatile connectivity.
- Analog Features:
- 10-bit ADC with up to 5 channels
- Internal voltage reference for enhanced analog precision
- GPIO:
- Multiple I/O pins with programmable pull-up, output type, and speed settings
- Up to 38 I/O ports (depending on the package)
- Operating Voltage: 2.95 V to 5.5 V
- Temperature Range: -40°C to +85°C (industrial-grade)
- Packaging: Available in LQFP32 and other compact package options.
Pin Configuration
The STM8S105K4 provides up to 48 I/O pins depending on the package, which can be configured for multiple functions such as ADC input, PWM output, UART, SPI, I²C, and general-purpose digital I/O. Key GPIOs include:
- Port A (PA0 to PA7): Configurable for digital I/O and alternate functions.
- Port B (PB0 to PB7): Primarily used for I/O with specific pins supporting alternate functions.
- Port C, D, and E: Support for additional I/O, analog input, and timer functions, including PWM generation.

Each pin can be configured individually for input or output, with support for both push-pull and open-drain modes. Additionally, the pins are ESD-protected and capable of high-drive outputs for LED and relay driving applications.
Block Diagram
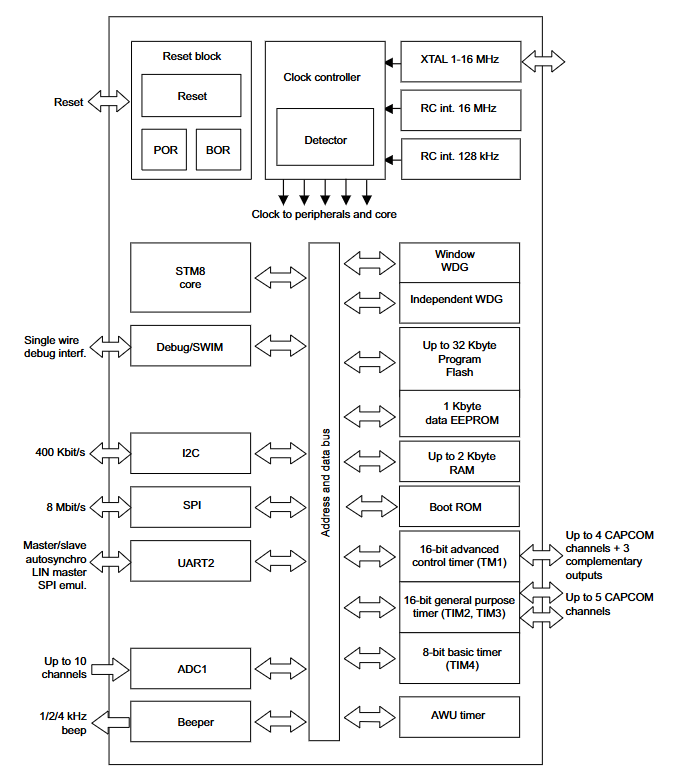
The block diagram of the STM8S105K4 includes:
- Core: STM8 core with clock control, program counter, and ALU for 8-bit processing.
- Memory Units:
- Flash memory for code storage
- EEPROM for data retention
- SRAM for general-purpose usage
- Peripherals:
- ADC for analog signal processing
- Timers (TIM1, TIM2, and TIM4) for event timing, PWM, and waveform generation
- Communication interfaces (UART, SPI, and I²C) for connectivity with sensors, displays, and other modules
- System Control:
- Clock generation unit with internal and external clock sources
- Watchdog timer for system reliability
- Power management unit with low-power modes
- I/O Control: GPIO configuration and management for interfacing with external devices
This modular architecture allows for flexibility in handling a variety of tasks, from real-time control to serial communication.
Applications
The STM8S105K4 microcontroller is ideal for a wide range of applications, including:
- Consumer Electronics: Home appliances, remote controls, and display control
- Industrial Control: Motor control, HVAC systems, and PLC modules
- Automotive Applications: Sensor interfacing, dashboard control, and lighting systems
- Healthcare: Medical devices, monitoring systems, and portable health equipment
- IoT Devices: Smart sensors, wireless modules, and energy metering
With its mix of analog, digital, and communication features, the STM8S105K4 enables designers to create efficient, versatile, and cost-effective embedded systems across various industries.
Creating an IAR Project for STM8S105K4
In this example, we’ll create an IAR project to light up the led using the STM8S105K4 microcontroller.
Required Tools
Hardware Components:
- STM8S105K4 Microcontroller
- ST-LINK/V2 Debugger and Programmer
- STM8S105K4 Development Board (optional)
- LED and Resistor (1KΩ)
- Breadboard and Jumper Wires
Software Tools:
- IAR Embedded Workbench for STM8
- ST Visual Programmer (STVP)
Steps to Create the Project
Create Project Folder:
- Create a folder named
test
, and within it, create another folder nameduser
.
- Create a folder named
Open IAR Embedded Workbench:
- Open IAR for STM8 (version 9.40.2).
Create a New Project:
- Go to
Project
->Create New Project
. - In the dialog box, select
STM8 Series
->Empty project
, and clickOK
. - Save the
.ewp
file in thetest/user
folder and name ittest
.
- Go to
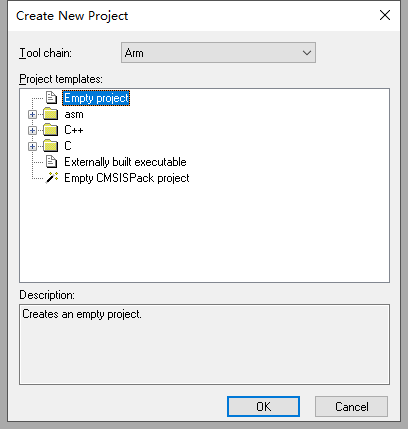
Add Project Group:
- Target the menu and click
Project
->Add Group
. - Name the group
user
, and clickOK
.
- Target the menu and click
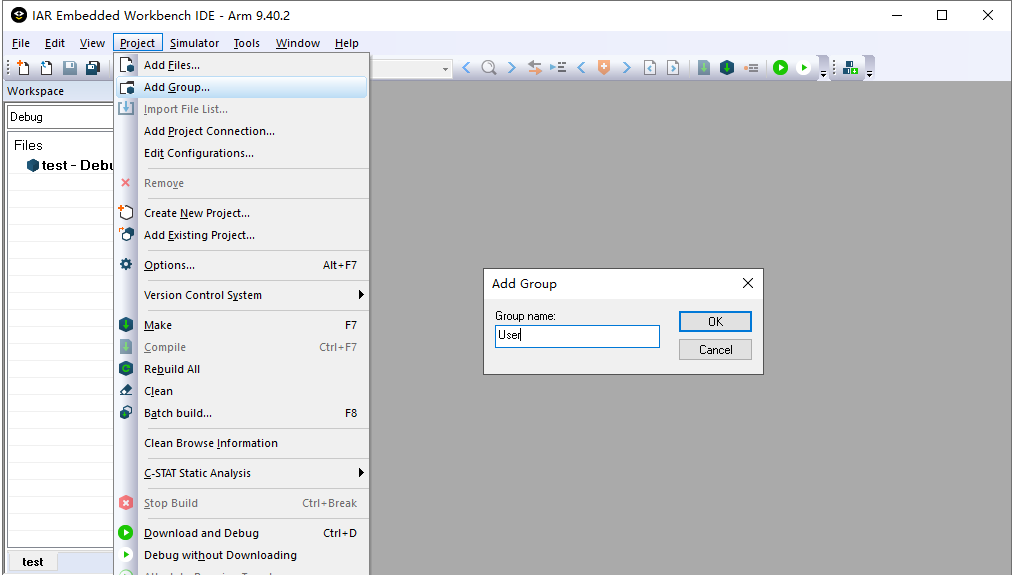
Create a Main File:
- Go to
File
->New
->File
, then save it asmain.c
. - Add
main.c
to theuser
group.
- Go to
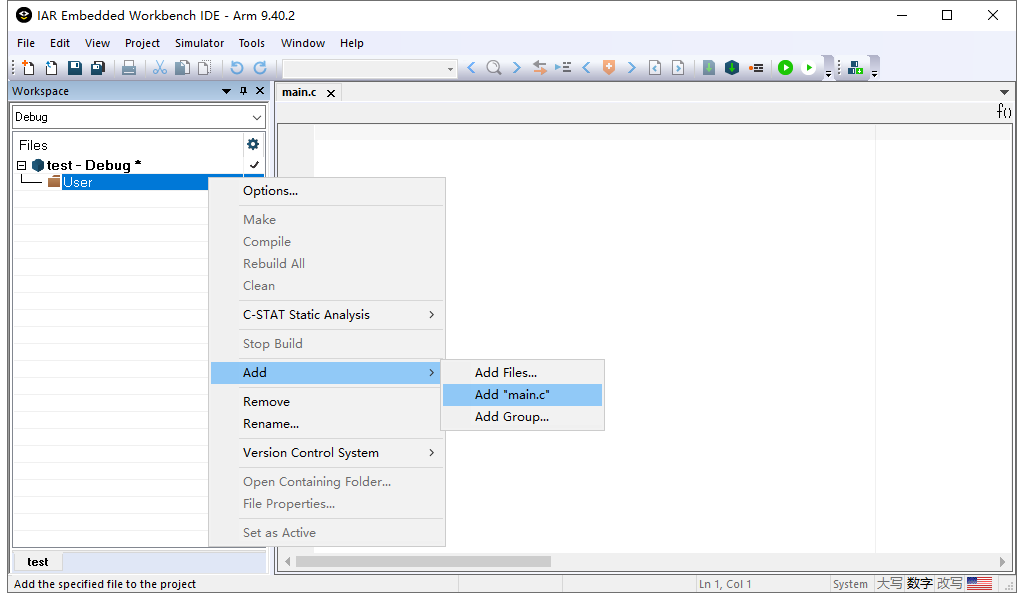
Configuring the IAR Environment
Project Options:
- Target and click
Project
->Options
.
- Target and click
Set Target Device:
- In
General Options
->Target
->Device
, selectSTM8S105K4
(or your specific device model).
- In
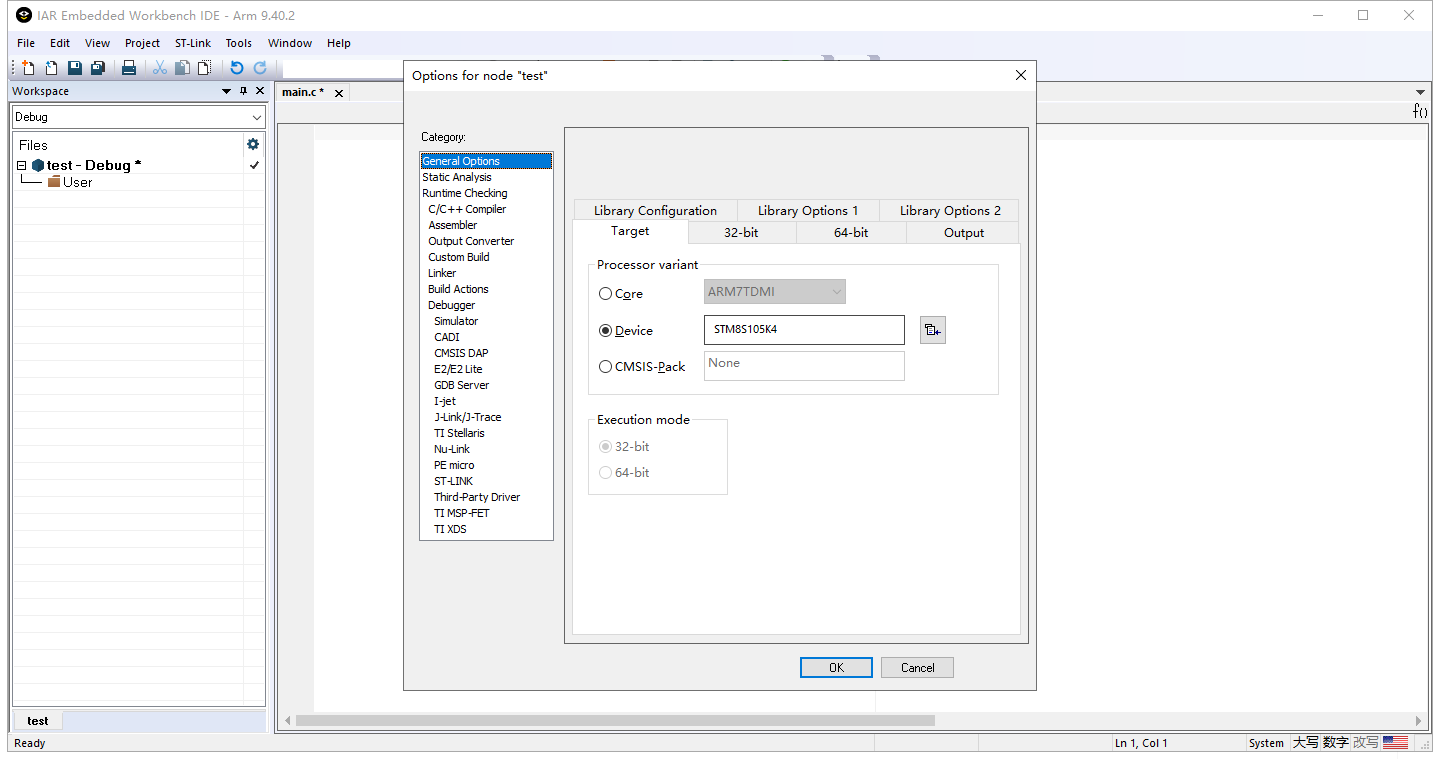
Configure Include Paths:
- In
C/C++ Compiler
->Preprocessor
, add the path"$PROJ_DIR$\..\user"
. - This syntax specifies the include file path within the project directory.
- In
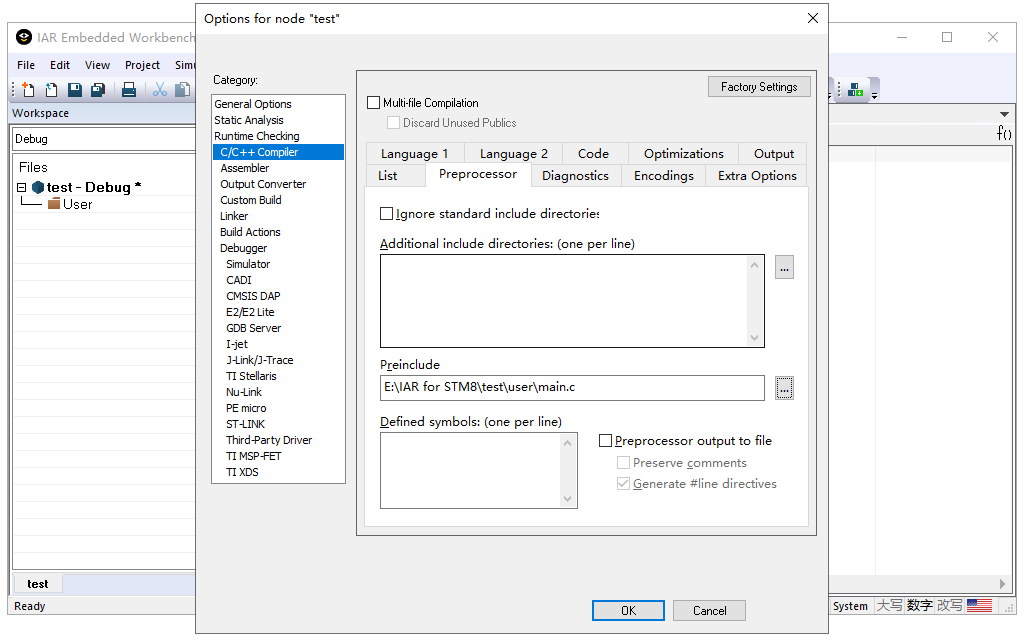
Setup Debugger:
- In
Debugger
->Setup
, set theDriver
toST-LINK
. - Click
OK
to save the configuration.
- In
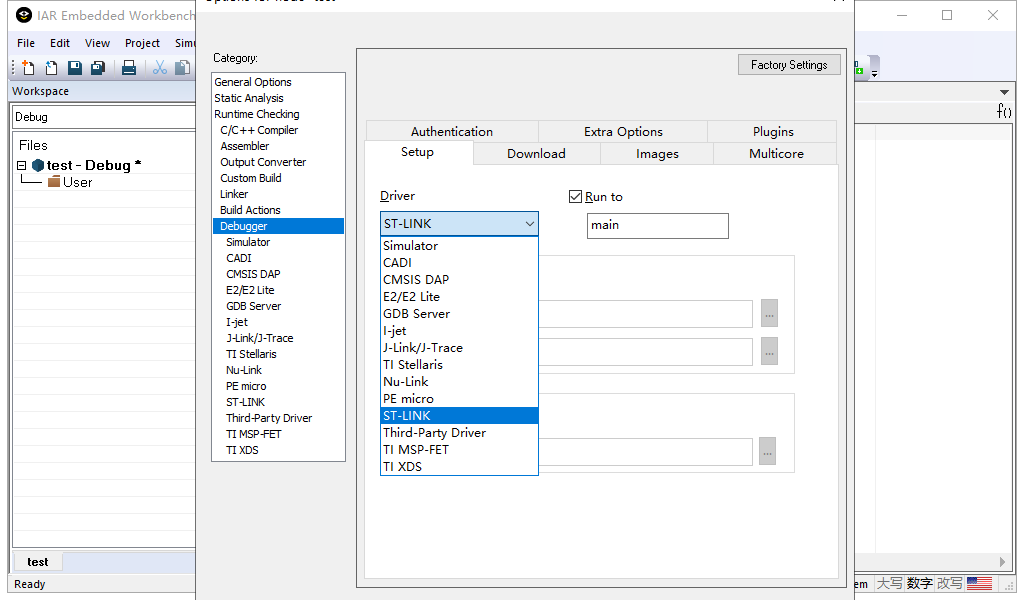
Add Code and Build the Project:
- In
main.c
, enter the following code, then go toProject
->Rebuild All
. - If you see
Total number of errors: 0
andTotal number of warnings: 0
, the project is set up correctly.
- In
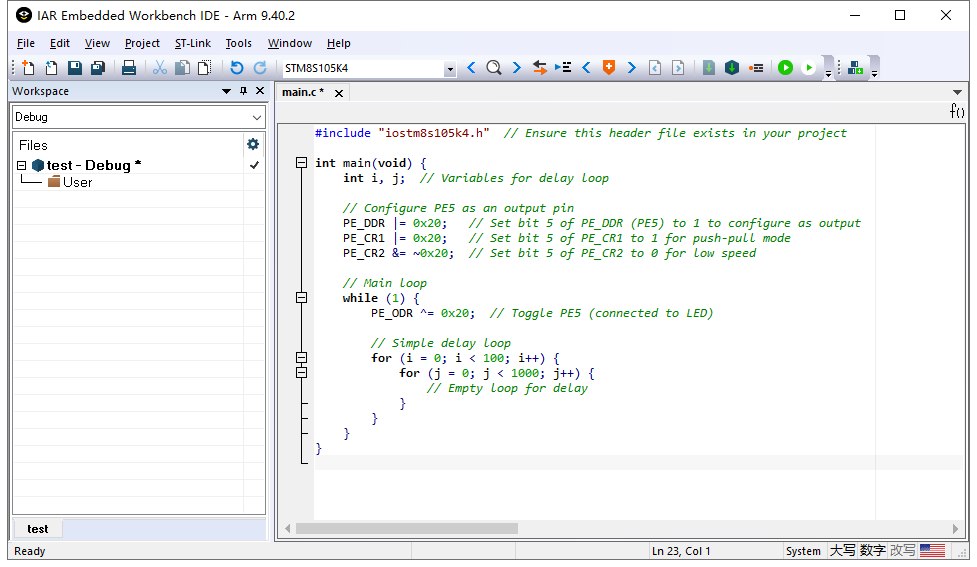
Include Header File:
- Ensure the header file
IOSTM8S105K4.h
is available in the IAR installation directory:Software (E:) > IAR for STM8 > arm > inc > ST
.
- Ensure the header file
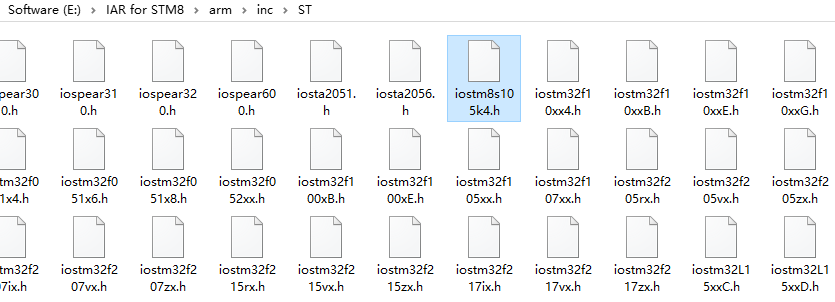
Writing, Downloading, and Debugging Code
The following code toggles an LED connected to pin PE5 with a delay loop to make the LED blink.
#include "iostm8s105k4.h" // Ensure this header file exists in your project
int main(void) {
int i, j; // Variables for delay loop
// Configure PE5 as an output pin
PE_DDR |= 0x20; // Set bit 5 of PE_DDR (PE5) to 1 to configure as output
PE_CR1 |= 0x20; // Set bit 5 of PE_CR1 to 1 for push-pull mode
PE_CR2 &= ~0x20; // Set bit 5 of PE_CR2 to 0 for low speed
// Main loop
while (1) {
PE_ODR ^= 0x20; // Toggle PE5 (connected to LED)
// Simple delay loop
for (i = 0; i < 100; i++) {
for (j = 0; j < 1000; j++) {
// Empty loop for delay
}
}
}
}
Hardware Connections
- PE5 → LED → 1K Resistor → Ground
After uploading the code and starting the program, you should see the LED on PE5 blinking, confirming that the project setup is complete.
Drive a Passive Beeper by STM8S105K4
In this example, the STM8S105K4 microcontroller’s pin PD4 is used to drive a passive beeper. The beeper function is an alternate function of PD4. By configuring the AFR7 bit, we can enable the alternate function for PD4, which allows it to drive the beeper.
Code Execution
/* Includes */
#include "user.h"
/* Function Prototypes */
void HalBeep_Init(BEEP_Frequency_TypeDef beep_fre);
/* Main Function */
void main(void)
{
/* Clock, LED, and Timer Initialization */
HalCLK_Config();
HalLed_Init();
HalTimer1_Init();
// HalUART2_Init(); // Uncomment if UART is required
/* Buzzer Initialization */
HalBeep_Init(BEEP_FREQUENCY_2KHZ); // Initialize the buzzer at 2kHz
BEEP_Cmd(ENABLE); // Enable the buzzer
enableInterrupts(); // Enable interrupts
while (1)
{
// Main loop - insert additional code here if needed
}
}
/* Buzzer Initialization Function */
void HalBeep_Init(BEEP_Frequency_TypeDef beep_fre)
{
BEEP_DeInit(); // Reset the BEEP registers to their default values
BEEP_Init(beep_fre); // Initialize the BEEP with the specified frequency
}
Programming and Configuring the PD4 beeper Function
- Flash the Program using ST-Link and STVP Software:
- Use the ST-Link programmer and the official STVP software to flash the hex file onto the microcontroller.
- Steps for Flashing and Configuration in STVP:
- Step 1: Open the STVP software, select the “PROGRAM MEMORY” option, go to
File -> Open
, and locate the saved hex file. - Step 2: Select the “OPTION BYTE” option and modify the AFR7 bit to enable the alternate function for PD4.
- Step 3: In the software menu, go to
Program -> All Tabs
to flash both the program and option bytes.
- Step 1: Open the STVP software, select the “PROGRAM MEMORY” option, go to
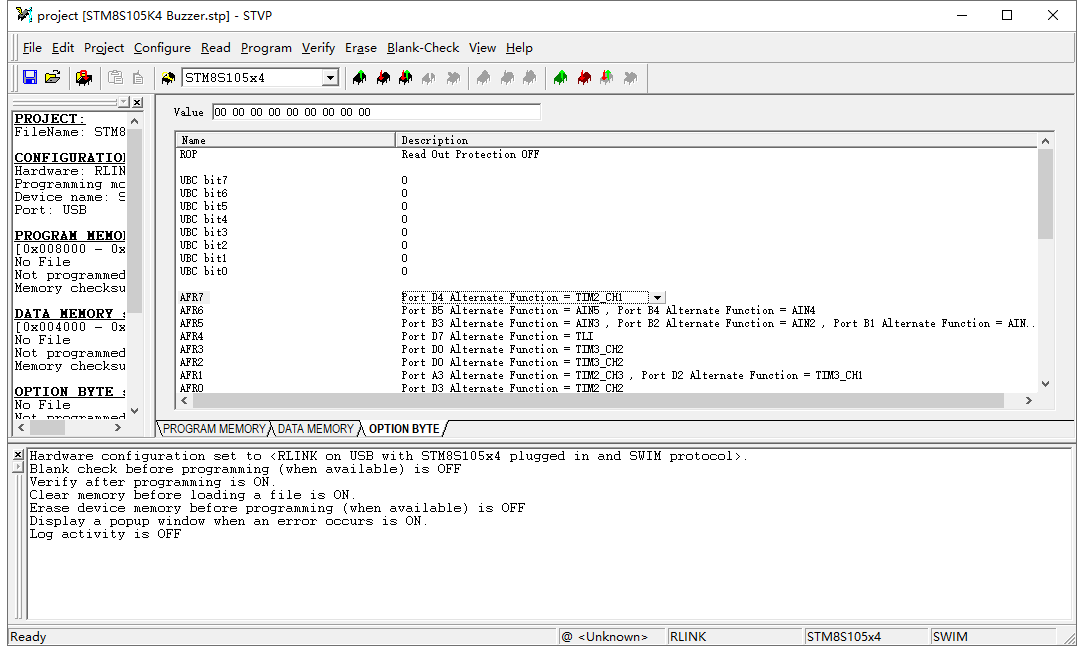